1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
|
# StudentDatabase
A website for comfortable collecting data about students.
## Spring
Project is based on Spring framework.
### Aplication
On start application checks if any roles in database, if there is not any, then creates `ADMIN` and `USER` roles.
```java
@Autowired
private RoleRepository roleRepository;
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Bean
CommandLineRunner init() {
return args -> {
saveRole("ADMIN");
saveRole("USER");
};
}
private void saveRole(String role) {
if (roleRepository.findByRole(role) == null) {
roleRepository.save(new Role(role));
}
}
```
### CustomAuthenticationSuccessHandler
If visitor is an user he will be redirected to home page, otherwise if visitor is an admin he will be redirected to admin panel.
```java
@Override
public void onAuthenticationSuccess(HttpServletRequest request, HttpServletResponse response, Authentication authentication) throws IOException {
response.setStatus(HttpServletResponse.SC_OK);
for (GrantedAuthority auth : authentication.getAuthorities()) {
switch (auth.getAuthority()) {
case "USER" -> response.sendRedirect("/");
case "ADMIN" -> response.sendRedirect("/admin-panel/information/students");
}
}
}
```
### WebSecurityConfig
Spring Security secures your password by `BCryptPasswordEncoder` method.
```java
@Autowired
private BCryptPasswordEncoder bCryptPasswordEncoder;
@Autowired
CustomAuthenticationSuccessHandler customAuthenticationSuccessHandler;
@Bean
public UserDetailsService mongoUserDetails() {
return new CustomUserDetailsService();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
UserDetailsService userDetailsService = mongoUserDetails();
auth
.userDetailsService(userDetailsService)
.passwordEncoder(bCryptPasswordEncoder);
}
```
### AdminPanelController
Application creates student accounts by given arguments.\
Example: 310-20 (`group`) and 10 (`number`). It will create 10 accounts with default login and password for 310-20 group students.
```java
@GetMapping("/admin-panel/create-users")
public String createUsers(@RequestParam String group, @RequestParam int number, Map<String, Object> model) {
for (int i = 1; i <= number; i ++) {
Role userRole = roleRepository.findByRole("USER");
User user = new User(i + "student" + group, i + "student" + group, true, new HashSet<>(List.of(userRole)));
User userFromDb = userRepository.findByUsername(user.getUsername());
if (userFromDb != null) {
model.put("message", "User exists!");
return "/admin-panel/add-users";
}
userDetailsService.saveUser(user);
}
return "redirect:/admin-panel/all-users";
}
```
## Gradle
Project uses Gradle as build automation tool.
Dependencies section in `build.gradle` file:
```gradle
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-data-mongodb:2.5.6'
implementation 'org.springframework.boot:spring-boot-starter-security:2.5.6'
implementation 'org.springframework.boot:spring-boot-starter-thymeleaf:2.5.6'
implementation 'org.springframework.boot:spring-boot-starter-web:2.5.6'
implementation 'org.springframework.boot:spring-boot-starter-tomcat:2.5.6'
testImplementation('org.springframework.boot:spring-boot-starter-test:2.5.6') {
exclude group: 'org.junit.vintage', module: 'junit-vintage-engine'
}
testImplementation 'org.springframework.security:spring-security-test:5.5.2'
}
```
## Screenshots
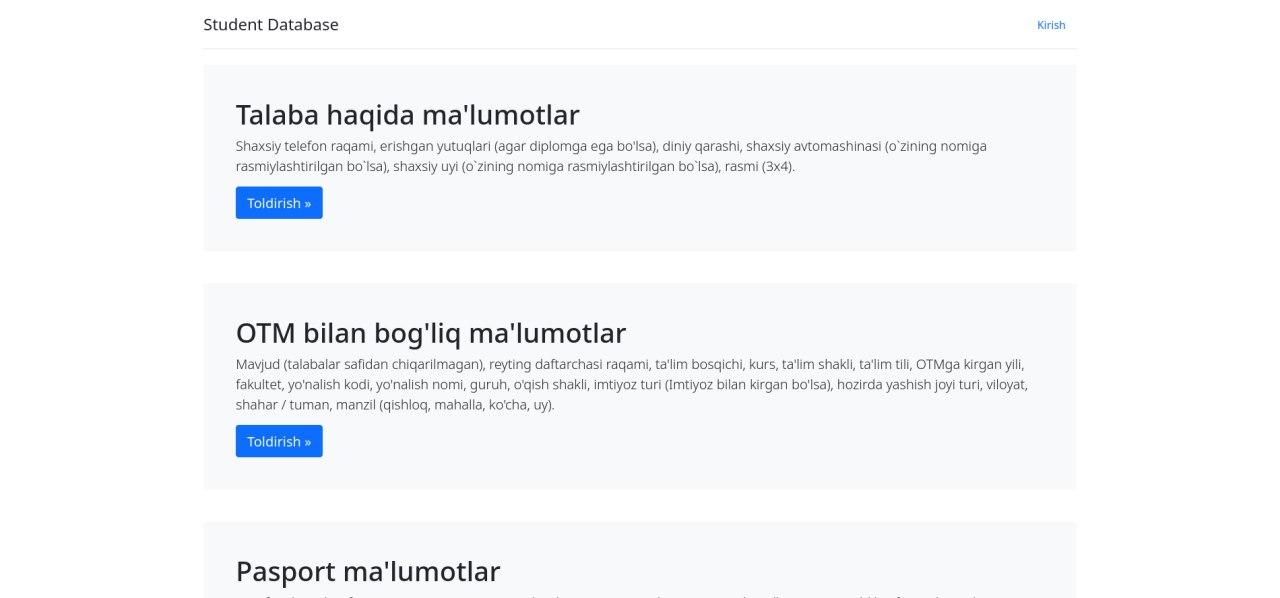
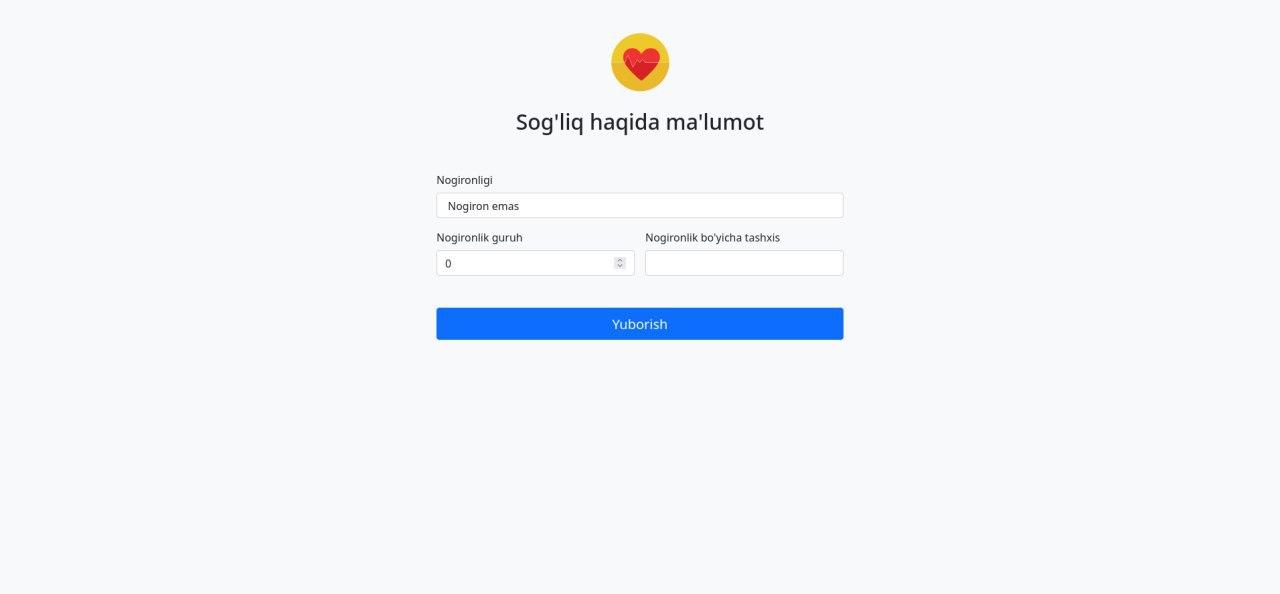
|